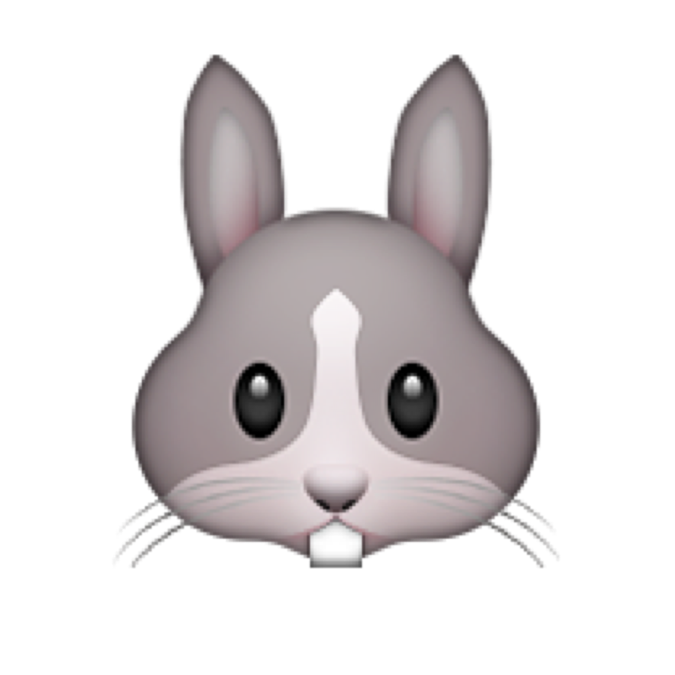
Emoji-Libs
My Challenge
Emoji-Libs was my final project for my front-end web development class. It's part of my design portfolio because it was a unique opportunity to create content, design, and actually bring a site to life with code. Of all the projects in my portfolio, this did the most to increase my understanding of the big picture, all the roles within tech, and how design can best work within them.
My Role
This project was 100% my work product, including concept creation, content, design, and code. Throughout the process our TAs were available to help and 5+ users provided feedback for concept and design.
My Process
Dos
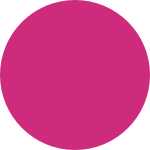
Must have JavaScript
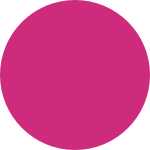
Must have some sort of animation
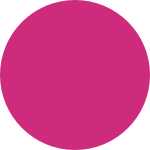
Could use APIs
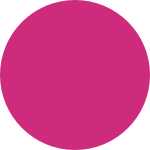
Could use Firebase
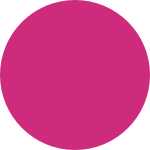
Quality over quantity
Don'ts
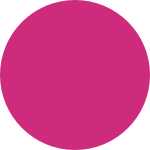
Use a project for work
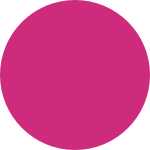
Use your portfolio for the final project
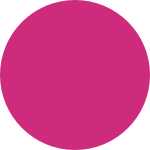
Present something you don't truly understand
Brainstorm Ideas
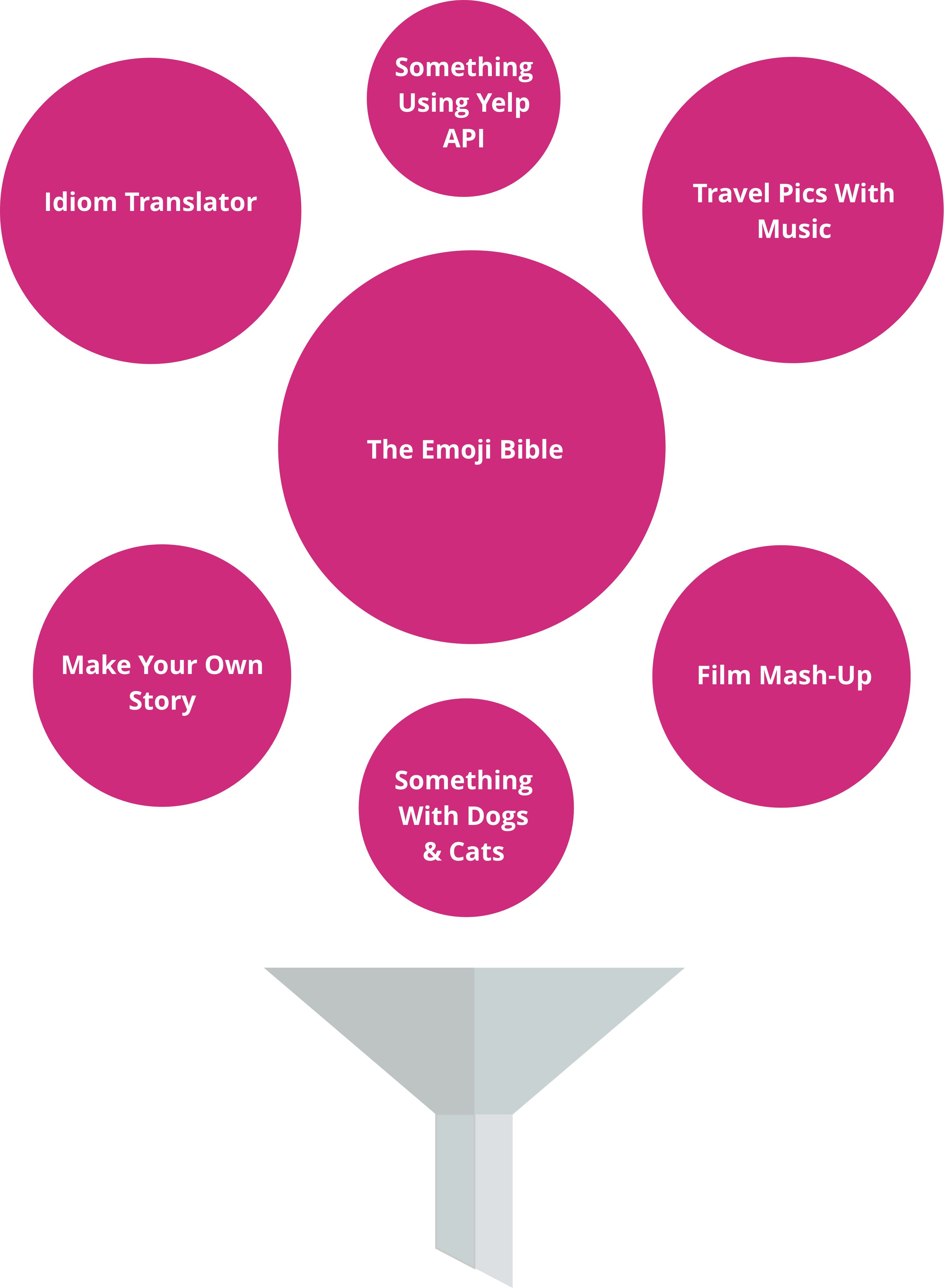
The Emoji Bible
Learn how to use emojis in three different ways: emoji-libs, express your feelings with emojis, and say things you have always wanted to say in emojis.
Idiom Translator
Translates English idioms into Spanish using Google Translate API. Also has animated cat that slides in after each idiom translation & says "Ole".
Travel Pics & Music
Shows travel pics from anywhere in the world with accompanying local music. Uses Instagram tags and Spotify and Instagram APIs
Vetting ideas
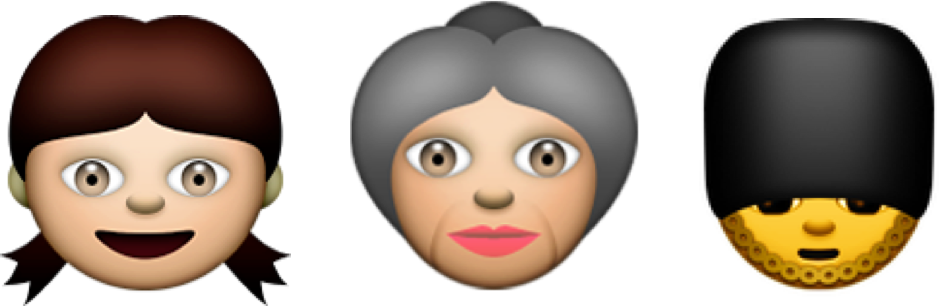
Concept user research
- Tested my top 3 ideas with 5+ users, ages 30-70
- Overwhelming favorite: The Emoji Bible
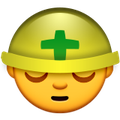
Reviewed ideas with engineers
- Discouraged me from using certain APIs, like Yelp
- Not excited about the travel site concept
- Liked the idiom translator
- Thought all my ideas were technologically viable
- However... "Emojis are just cool. You can't beat emojis!"
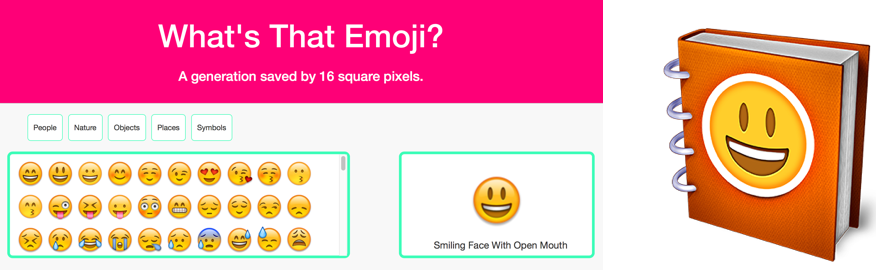
Competitor sites not too compelling
- Few sites dealt with understanding the meaning of emojis
- No sites offered Mad Libs with emojis
- Quality, design, and functionality of these sites were poor
Think big, start small
I chose the Emoji Bible, but did I bite off more code than I could chew? Our TAs said to cut back.
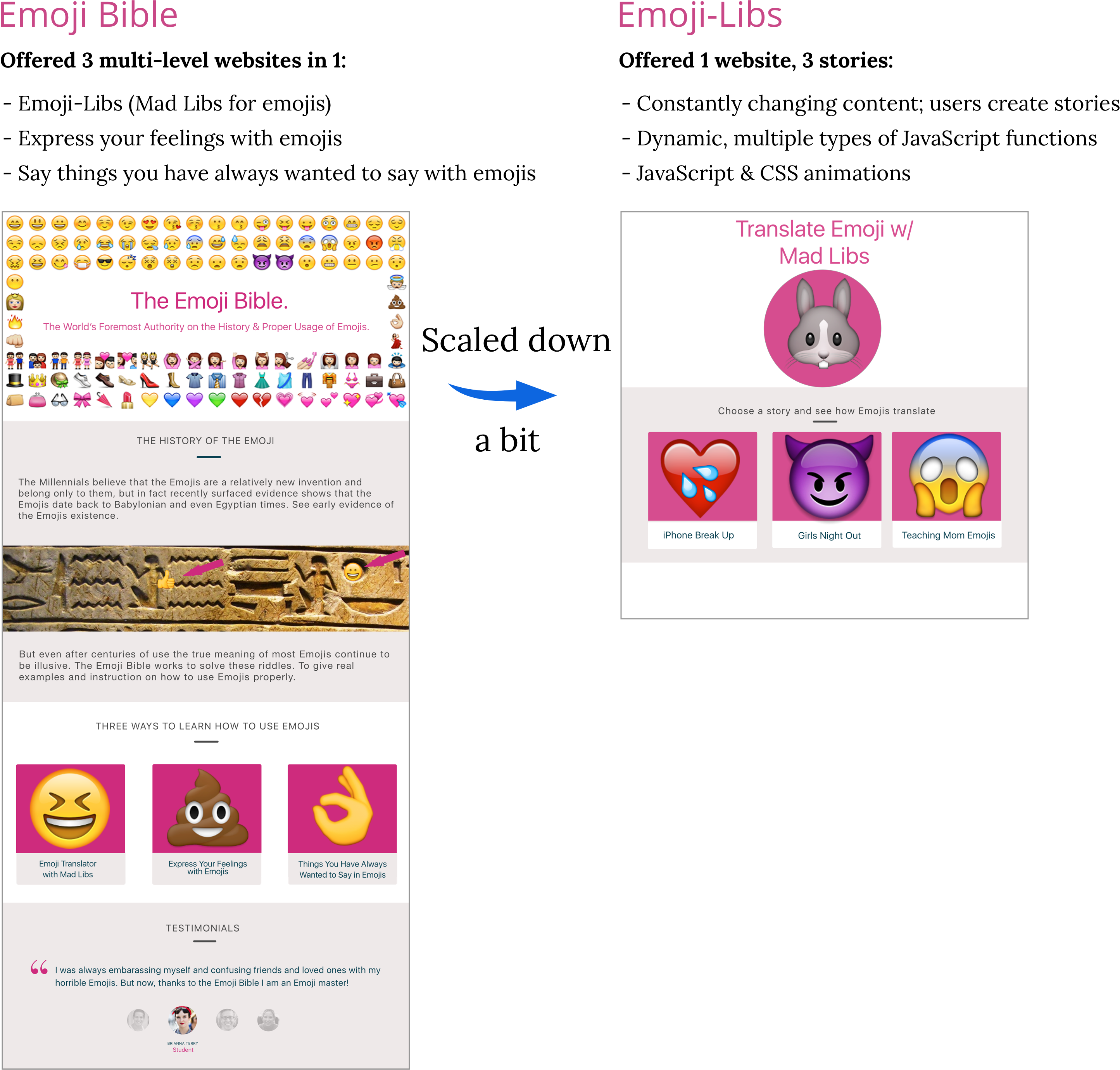
Where to start: the plan
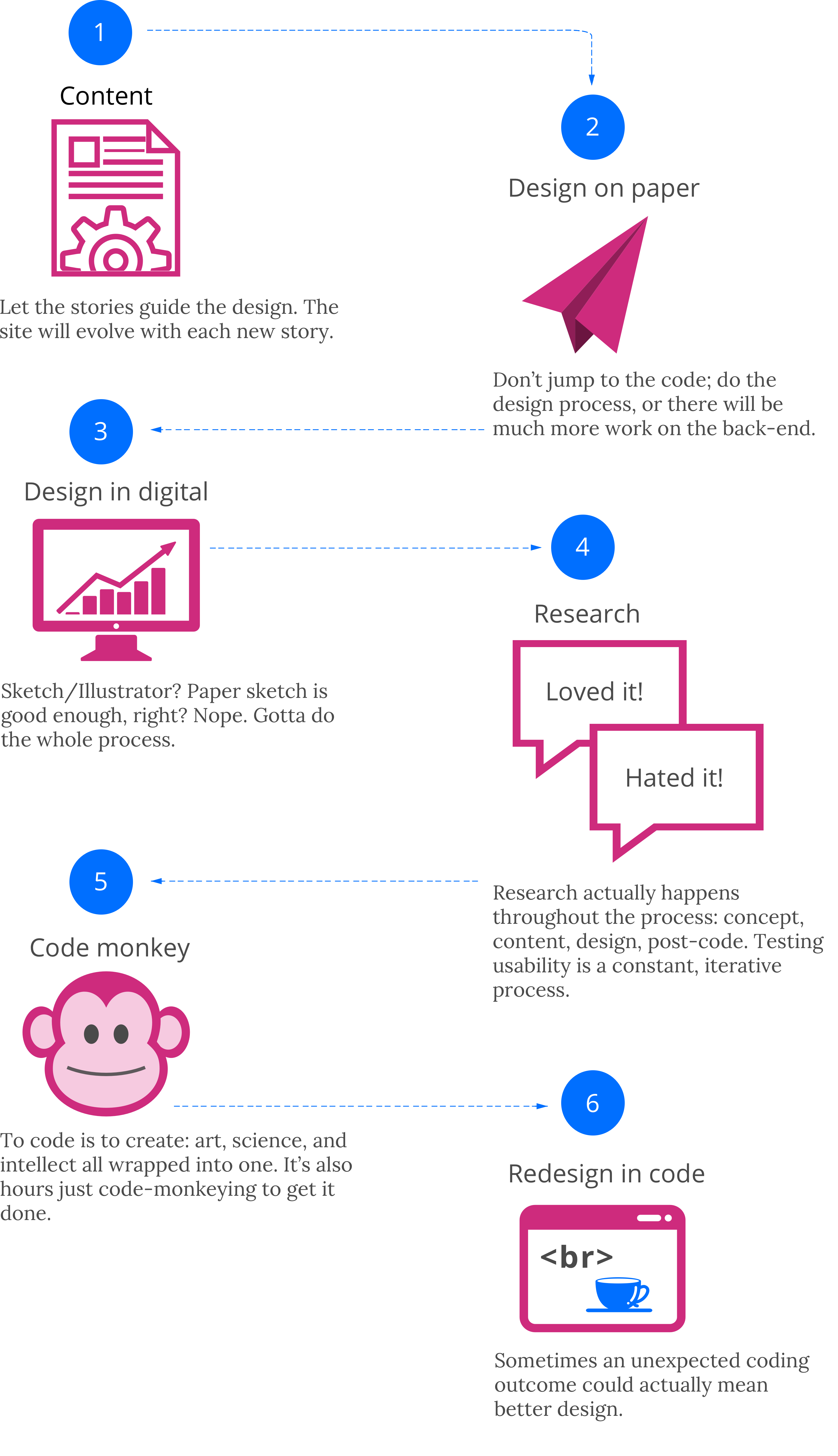
They say code is akin to language. I speak 2.25 languages and have taught language. I believe this analogy holds up, to a point. Having no coding experience prior to this class, I was learning more than a new language. I was learning an entirely new paradigm: a new way to think, work, and communicate with my computer.
HTML: a skeleton made of Tupperware
When I started learning HTML, I would get this picture in my mind of Tupperware. I saw the "divs" as the containers of the content (photos, text, etc.), the space around the containers as the margin, and the space around the food/content inside these containers as the padding. I also like the metaphor of HTML as a skeleton that you attach content to, so to me, HTML is a skeleton made of Tupperware.
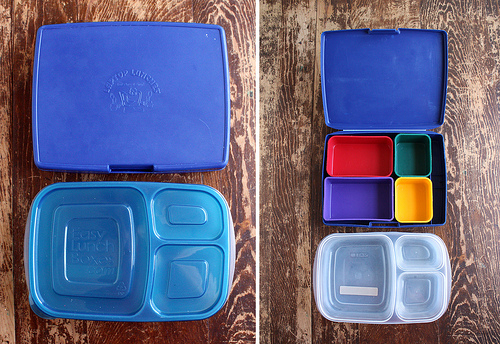
CSS is hard
My front-end development teacher, Avand, had this bad habit of saying, "That's easy." And like that annoying Staples "easy button," him saying it didn't actually make it so. For my final project, I was so focused on "making it work", I left CSS until the end. Avand said, "You can't save CSS to the end. CSS is hard!" This was a huge victory: Avand admited that something in code was hard! The truth is nothing in code can ever be taken for granted as "easy." Sometimes even the most basic things can trip you up.
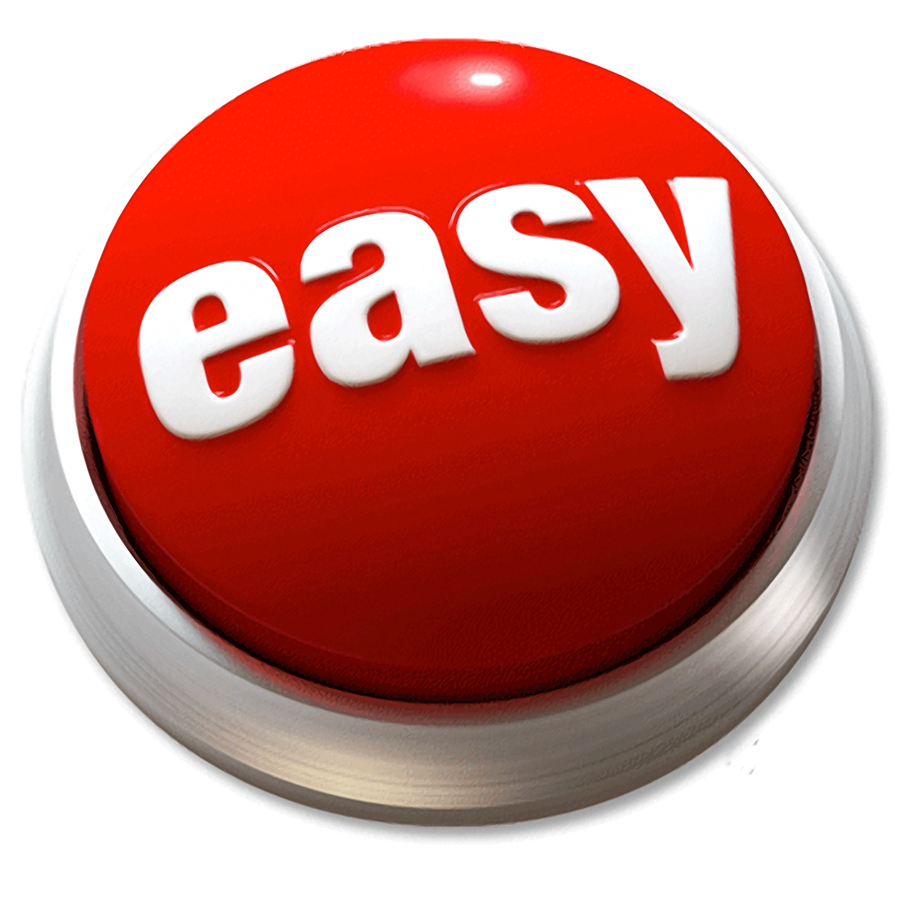
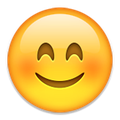
CSS is much more than a pretty face. It does much more than color and styling. For animations, many people even prefer CSS over JavaScript.
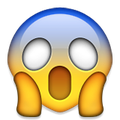
In CSS, he who speaks last, speaks loudest. This is a blessing and a curse. It's a blessing in that you can easily overwrite things by changing the order. But a curse as multiple classes (especially on elements: table, etc.) can cause you to accidently overwrite previous code.
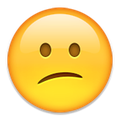
CSS may never give you "why" or "where." Some languages, like JavaScript, will tell you exactly where the error is and maybe even a bit about why it's not working. But with CSS you are mostly on your own. Good luck.
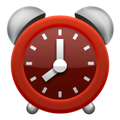
Time, time, time is not on your side. No it's not. Some of the seemingly "easiest" things in CSS can take hours, even for seasoned developers.
JavaScript is harder
And what's even worse: the analogies. Somehow visualizing variables as a "bucket" and functions as a "sausage factory" is supposed to help you to understand inherently difficult concepts. It doesn't.
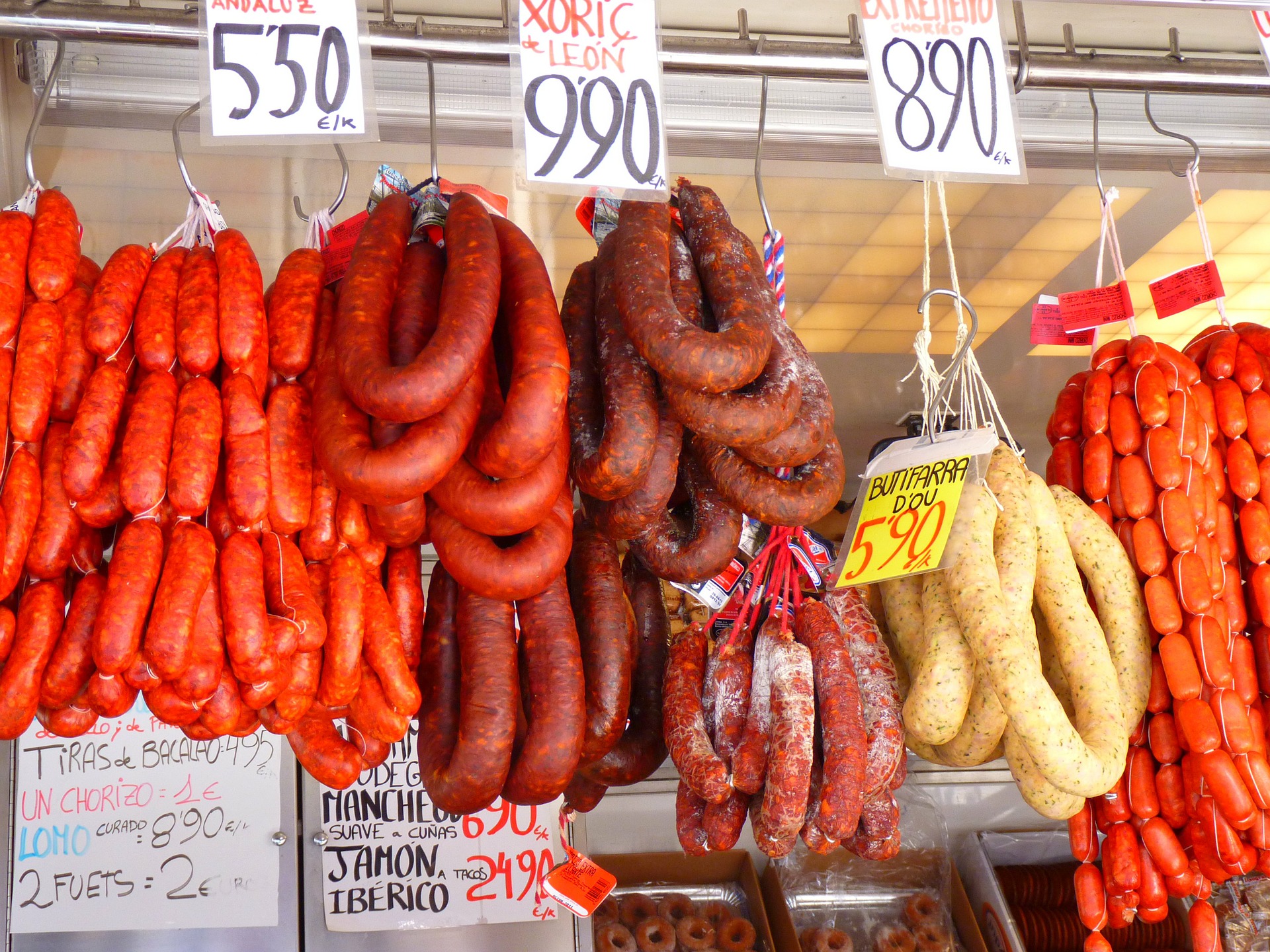
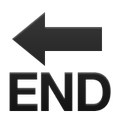
Everything shuts down. Unlike CSS/HTML, when JavaScript is not working every bit of your code just stops working, even parts that have nothing to do with the error. Good times. Good times.
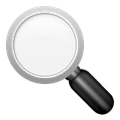
Gives you a clue about the "where" if not also the "why" (sort of). JavaScript tells you the exact line where the error starts. It also gives you information about what caused the error, although usually in computer speak: "Unexpected token ILLEGAL." Am I going to coding jail?
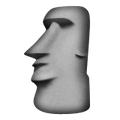
JavaScript is a very powerful tool. It was not lost on me that almost anything you want to do in front-end web development can be done in JavaScript.
Knowing some code is understanding all code
My new paradigm. I recently attended a Ruby pairing workshop. I had exactly zero experience in Ruby, or any other back-end code for that matter. I asked my "pair" the difference between Ruby and Ruby on Rails. I will paraphrase his answer in the style of the SAT: Ruby is to Rails as JavaScript is to jQuery. I had no experience with Ruby, but understood him completely. I know I have just cracked the surface, but learning any code going forward should be much easier given my new paradigm.
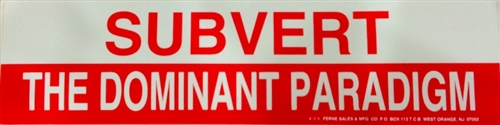
Computers are stupid, but never wrong
I can almost hear the collective groan from designers when they get to this part. "Oh no! No actual code!" I cut this tiny bit of code out of my Emoji-Libs site to demonstrate how I understand that computers think. Designed by mathematicians, computers "think" in a way that is not very obvious to most of us. There is a common phrase among coders: "Computers are stupid, but never wrong." This is to say that you have to tell computers what you want in explicit detail in a language that only they understand to get the output you want.
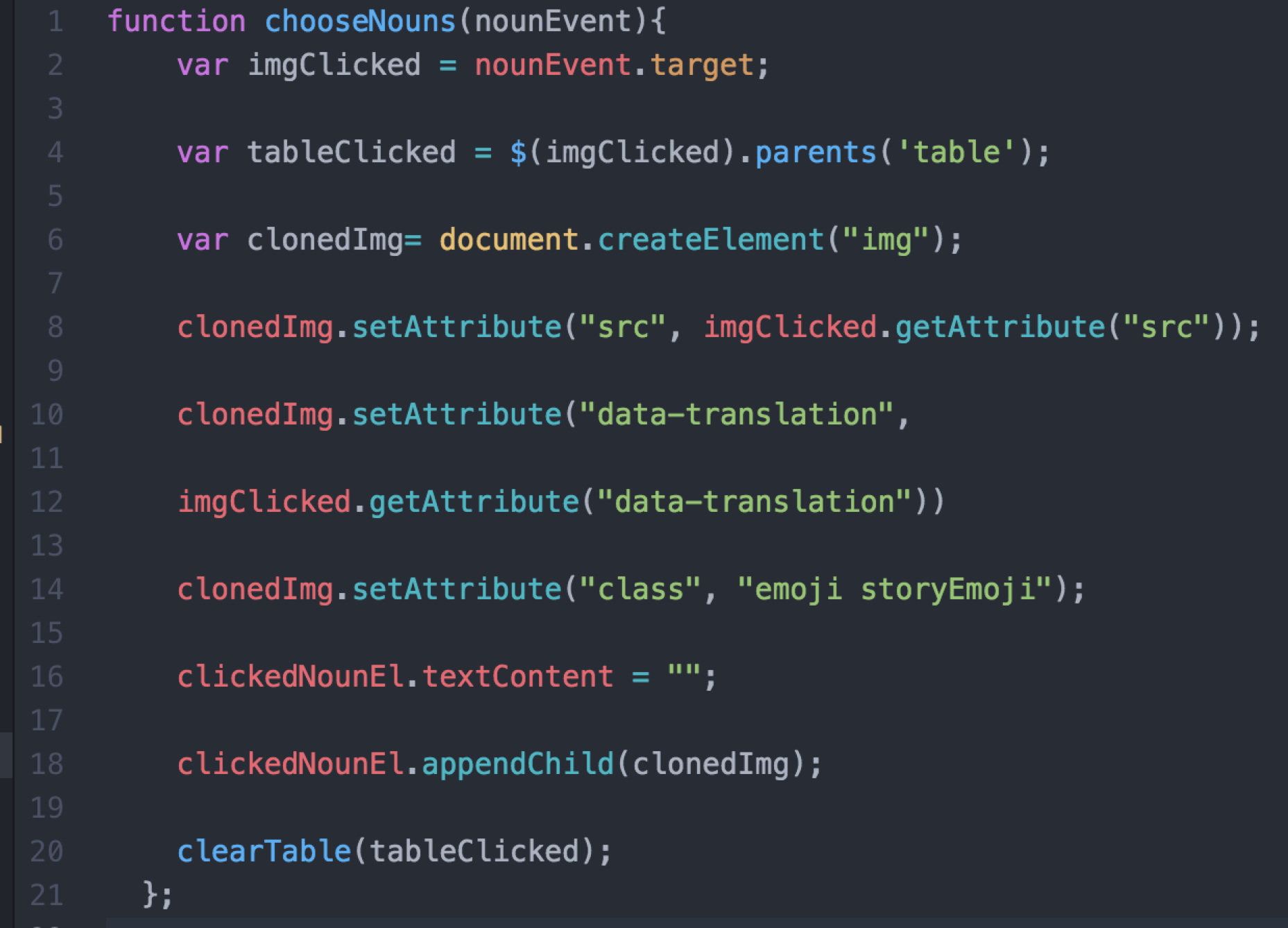
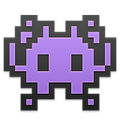
Line 1: function chooseNouns(nounEvent){
The function that allows you to choose a noun emoji from the list of options in the noun table.
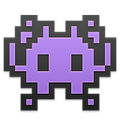
Line 2: var imgClicked = nounEvent.target;
The variable that remembers the emoji (shoe, etc.) you selected in the noun table so it can later be placed within the story text.
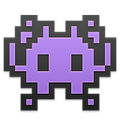
Line 4: var tableClicked = $(imgClicked).parents('table');
The variable that remembers that you are in the noun table so later you can clear the noun table.
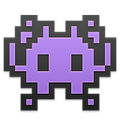
Line 6-14: var clonedImg= document.createElement("img");
This code creates a new emoji image to be placed in the text of the story and assigns it all the necessary attributes: image, classes, the translation text (i.e. pig emoji translates to the word "piggy").
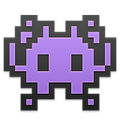
Line 16: clickedNounEl.textContent = "";
This code clears out the old content; in this case the word "noun" that was underlined in the text.
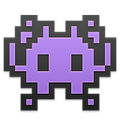
Line 18: clickedNounEl.appendChild(clonedImg);
That must be it, right? Nope. You actually have to tell the computer to append your new emoji image to the page so you can see it.
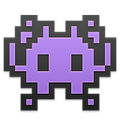
Line 20: clearTable(tableClicked);
Oh, and one more thing. Now you have to tell the computer to remove that table of nouns now that you don't need it anymore.
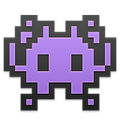
Phew. I am exhausted. And that was just one tiny piece of the code that makes up Emoji-Libs.
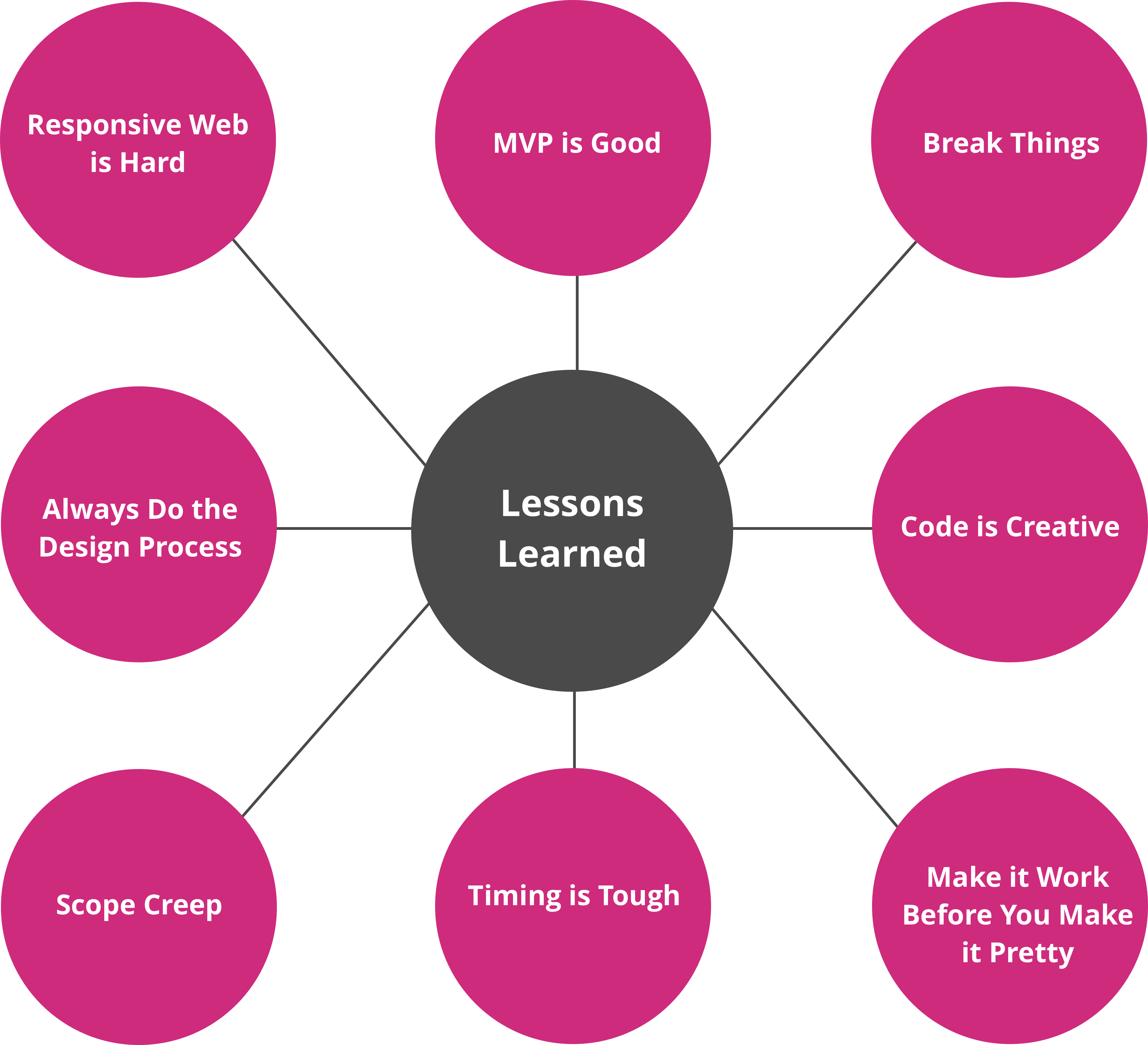
Break Things
- People don't want to change code for fear of breaking it, especially JavaScript.
- But the only way to learn is to change things, break things, and then fix them. (Commits on GitHub so you can "undo" are great too!)
Always Do the Process
- This was for FE Web Development. I didn't need to look at strategy, sketch designs, create wireframes, user test - any of that.
- But, for me, doing the design process is necessary for any kind of decision-making.
Make It Work, Before You Make It Pretty
- This phrase should be tattooed on the arm of any web developer or designer.
- True for design, for code it's especially true as the penalty for making it "pretty" too soon can be many hours/weeks wasted.
MVP is Good
- Focus on completing a MVP first - get it done.
- What is the minimum I need to do to get this out? The rest goes on your to do list. And it will be a long list.
- Also, the lean UX implied by MVP may also result in a crisper design.
Timing is Tough
- I learned a lot about what is easy/hard to do in code, but even veteran devs have problems like:
- The "easy CSS" issue that took 3 hrs to fix.
- The problem I didn't know I had.
- Just gonna finish one more thing… is it 4am?
Scope Creep
- Scope creep is the toughest thing to manage in code.
- I just need to do one more thing…
- Wouldn't it be cool if…
- STOP! Define the scope and stick to it. Don't let a small project become the Taj Mahal.
Responsive Web
- Bootstrap is helpful with nav, footer, etc., but requires work on visuals.
- Bootstrap's grid can help with layout, but you usually also need to insert media queries.
- Percentages and global margins make responsive easier.
Code is Creative
- Some think code is just being a "monkey," - rote implementation of someone else's creativity.
- For this project I created content, design, and code.
-And I found code to be very creative, often inspiring better design.The problem & the Emoji-Libs solution
Problem
People often get confused with the meaning of emojis and misuse them, leading to minor mix-ups and even relationship breakups.
Solution
Emoji-Libs gives you real examples so you can practice how to use Emojis properly, improving your Emoji Intelligence Quotient (or EIQ) dramatically overtime.
Initial concept & features
Translate emojis to real words
3 different stories you can do again & again
Make up your own story content
Cool
animations
User testing with paper prototypes
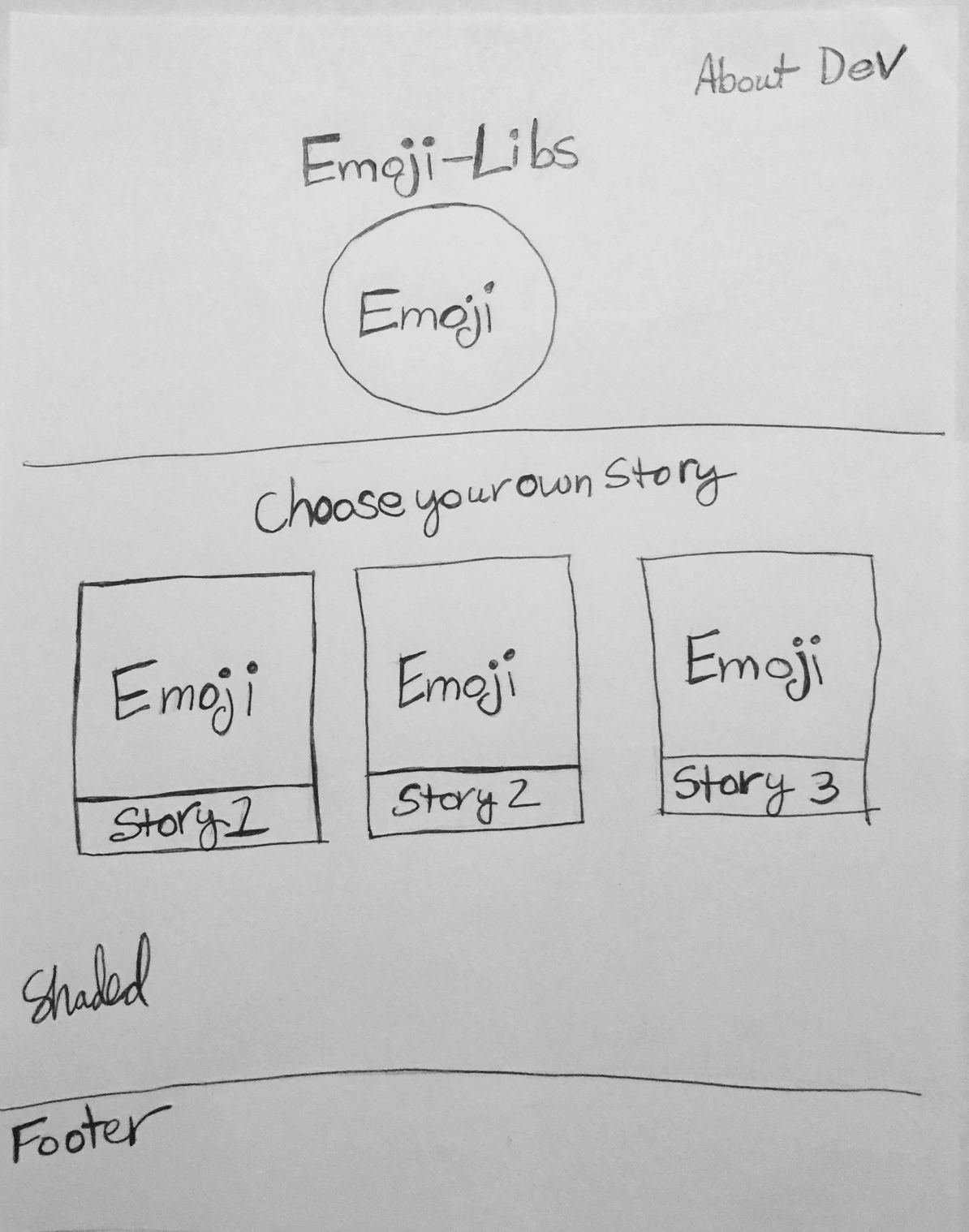
Emoji-Libs Home Page
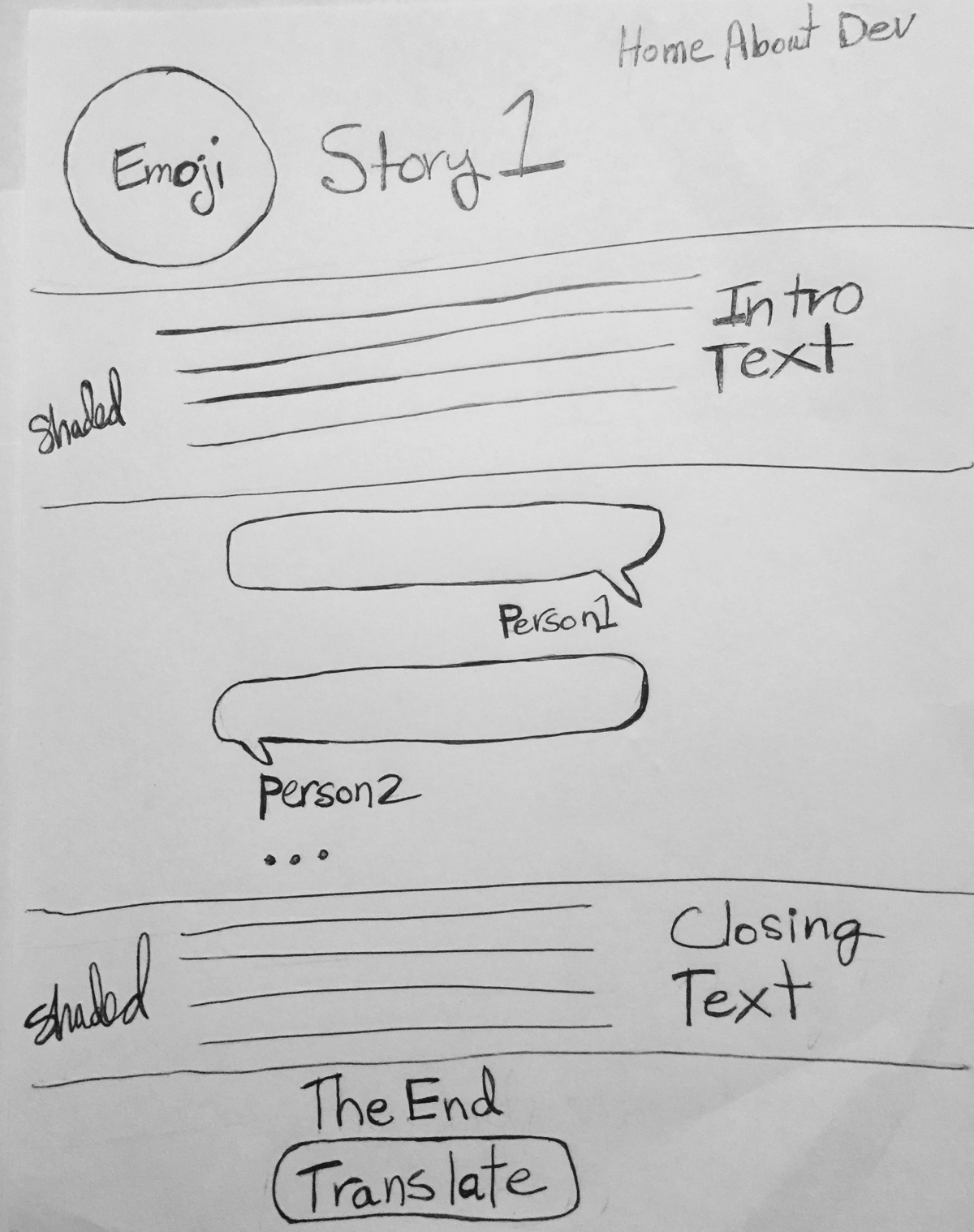
Sample Emoji-Libs Story
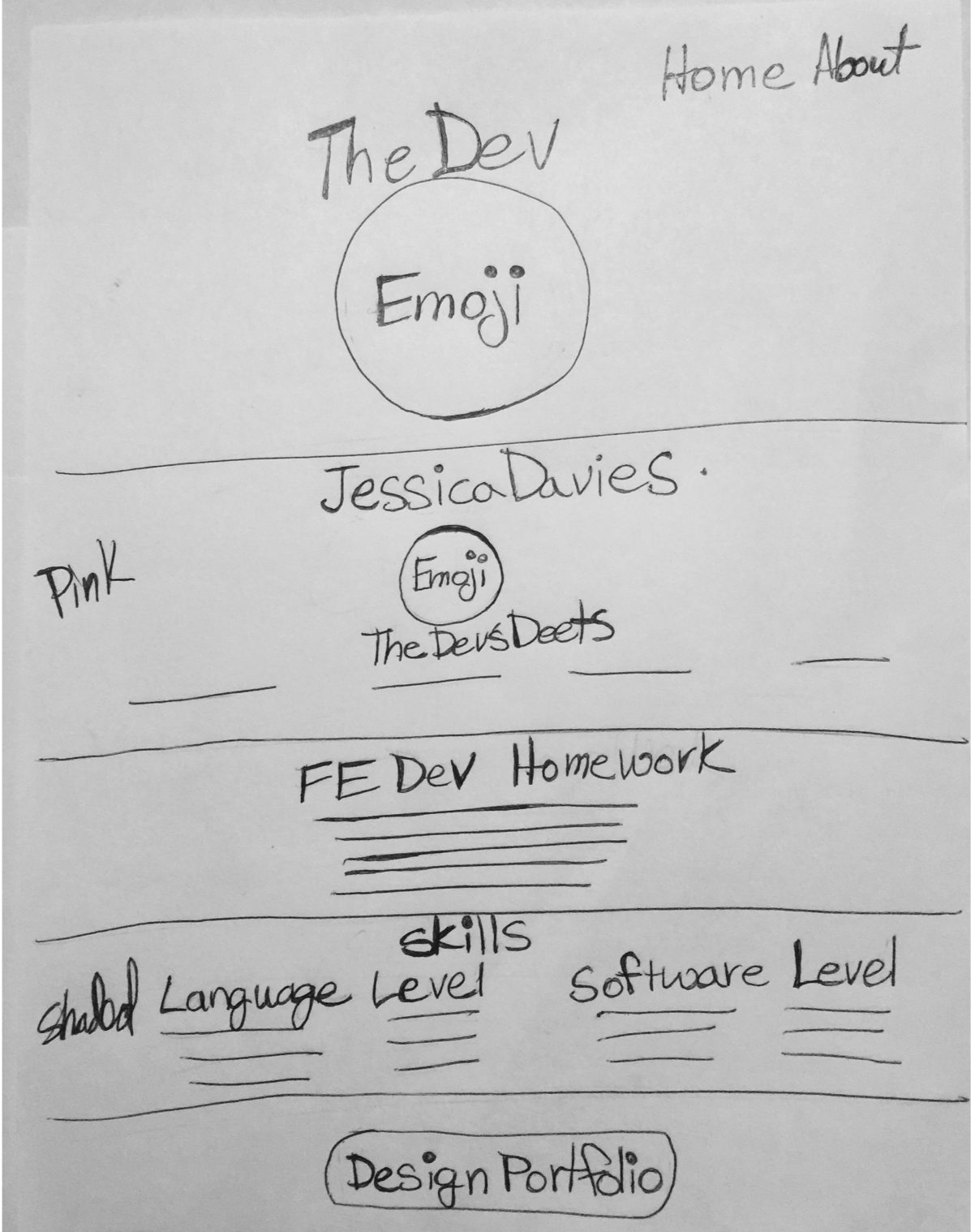
The Dev: Info About the Creator
Usability testing
User feedback improved UI design
Users saw the initial design as "clunky", going back and forth between blocks of text and cell phone texting bubbles. Due to their suggestions, I introduced the "monkey" narrator and that fixed the flow.
Users' prerogative to change their minds
Users mentioned that they might want to change their choice of emojis after they made a selection. I modified my JavaScript so they could make more than one choice.
Users loved the "History of the Emojis"
User's loved the "History of the Emojis section that appeared in the original draft of the Emoji Bible. Originally it was cut, but I found a way to repackage the content, using it for the Emoji-Libs' "About" page.
Added new section, "The Dev"
FE development projects, like most websites, typically have no info about the developer. A user was looking for a section with info on the site's creator. This gave me the idea to add "The Dev."
Why knowing code is important for designers
1
Understand the effort and why deadlines might be missed
Know what is easier to do in code and what might take more effort.
Understand that anything can happen makes you a better, more empathetic partner with developers.
2
Pitch in
In a start-up environment especially, it is important to be able to pitch in and do what you can to meet a deadline.
3
Knowledge is power
Sometimes dealing with engineers is like dealing with a mechanic: "Don't worry about what's under the hood, little missy."
When designers know code, they can take back some of that power.
3
Code can inspire/influence design
No matter how perfect the design, nothing renders quite the same on Sketch/Illustrator as it does on the web page.
Sometimes a coding accident or a need to modify the content to fit the code can lead to a better, cleaner design.
In addition to some of the general lessons learned about code and design, I also learned some "tricks" that made building my site easier. Keep in mind that some tricks or hacks, like using negative margin to move things up or margin left to center, can be very dangerous, especially when it comes to responsive web, and usually more trouble than they are worth. The tricks below are ones that have given me maximum speed/ease with minimum headaches. But with code you know I can't make any promises.
Tricks of the trade
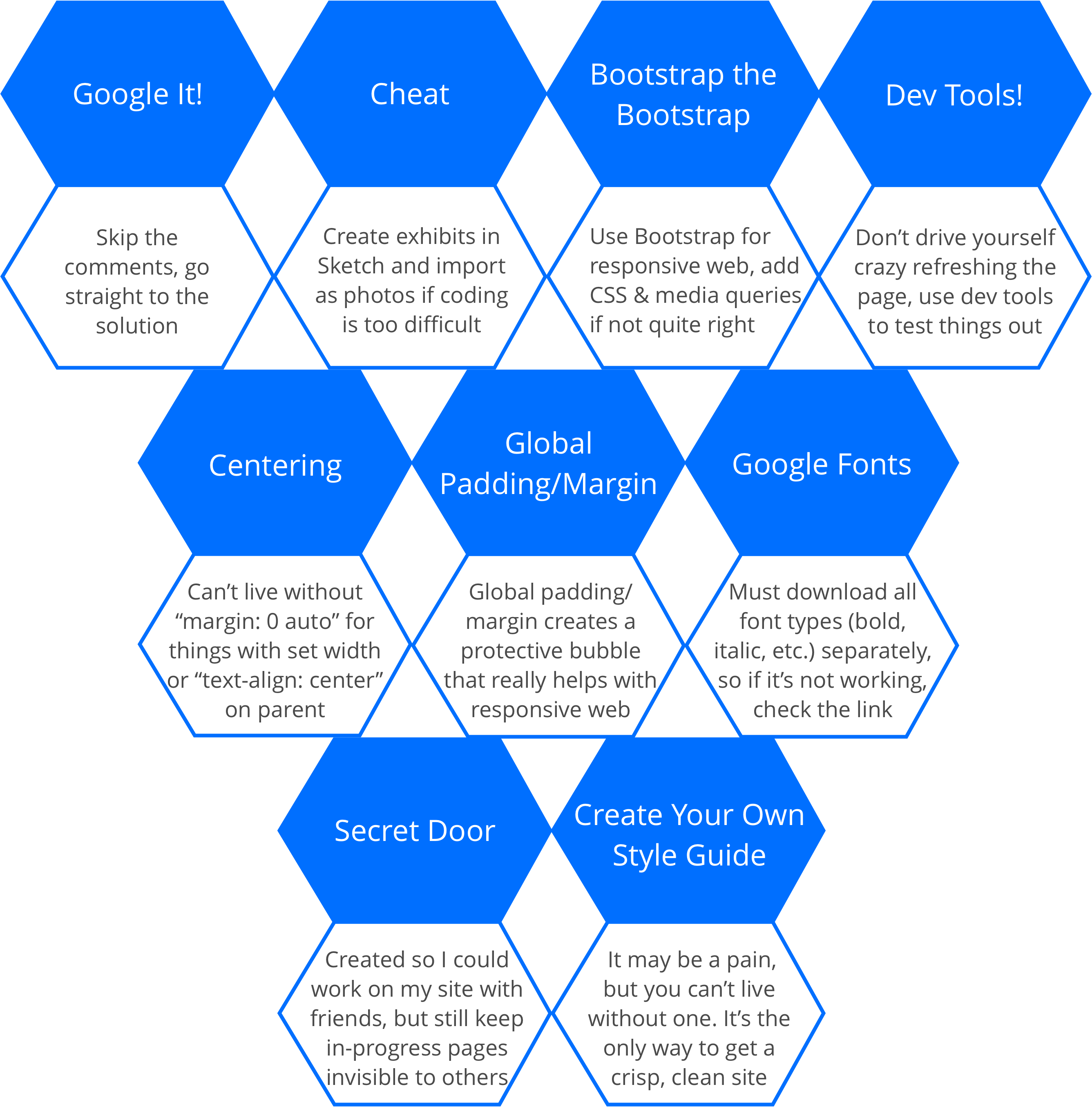
Emoji-Libs' features
Each story page offers a quick introduction/on-boarding
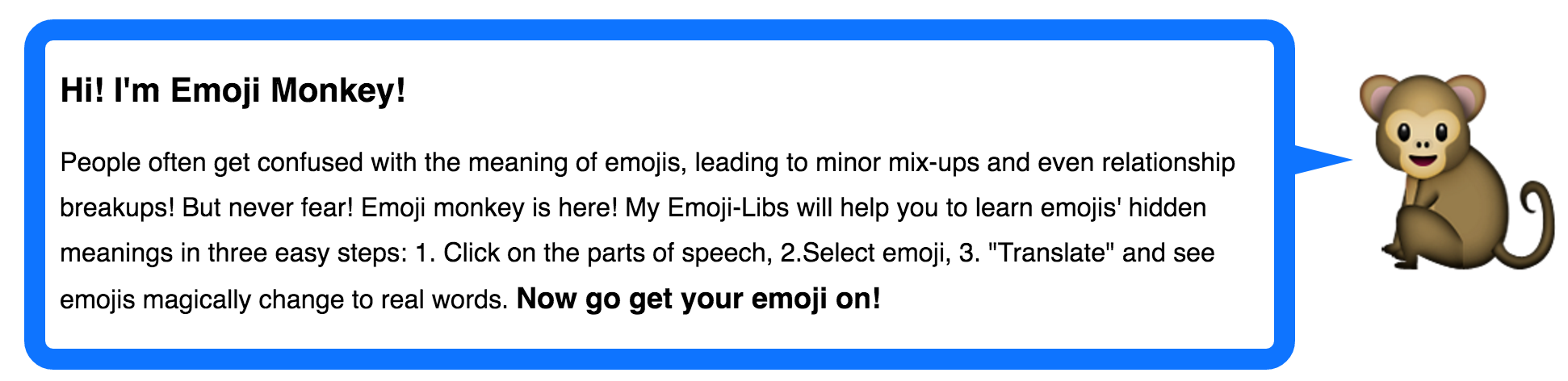
Bold, all caps, & underlined areas make it clear where users should click in the story
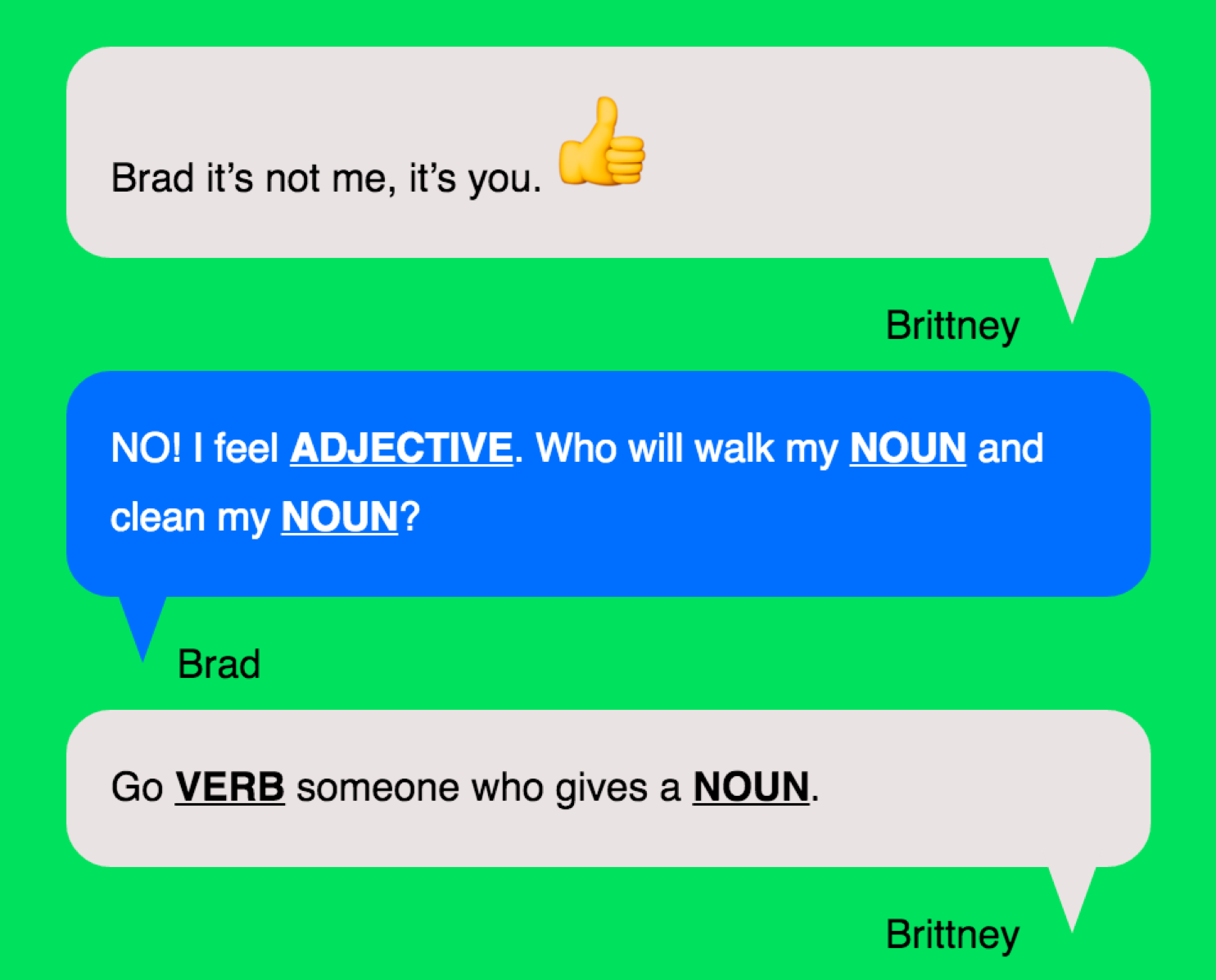
Easy-to-use pop-up table allows user to quickly choose correct emoji
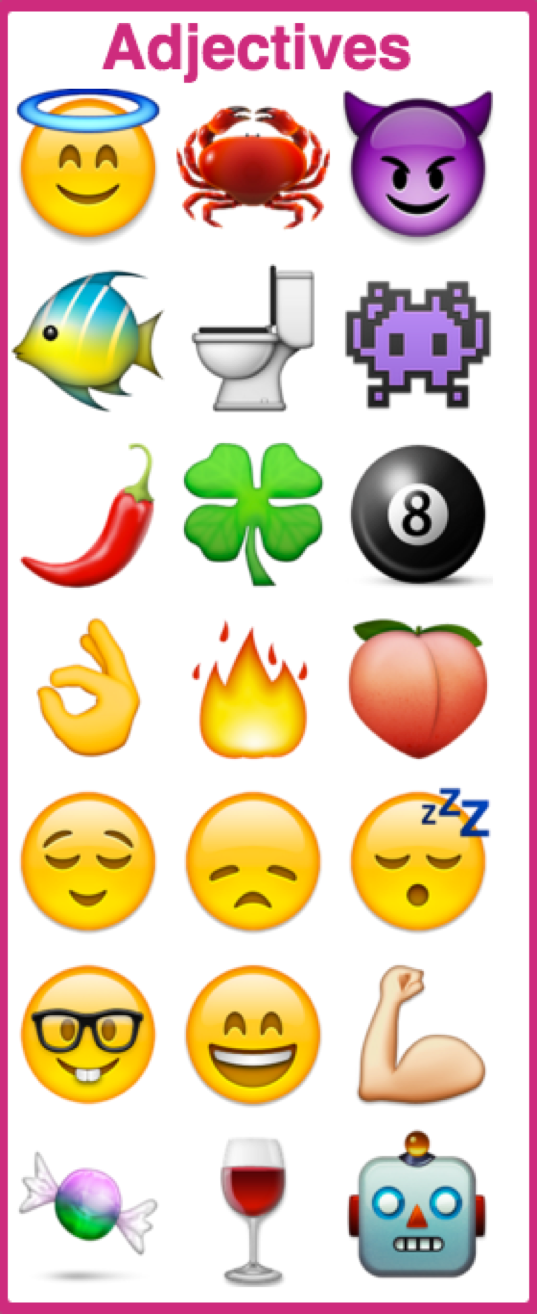
Hard to miss "translate" button lets user see how emojis translate to real words
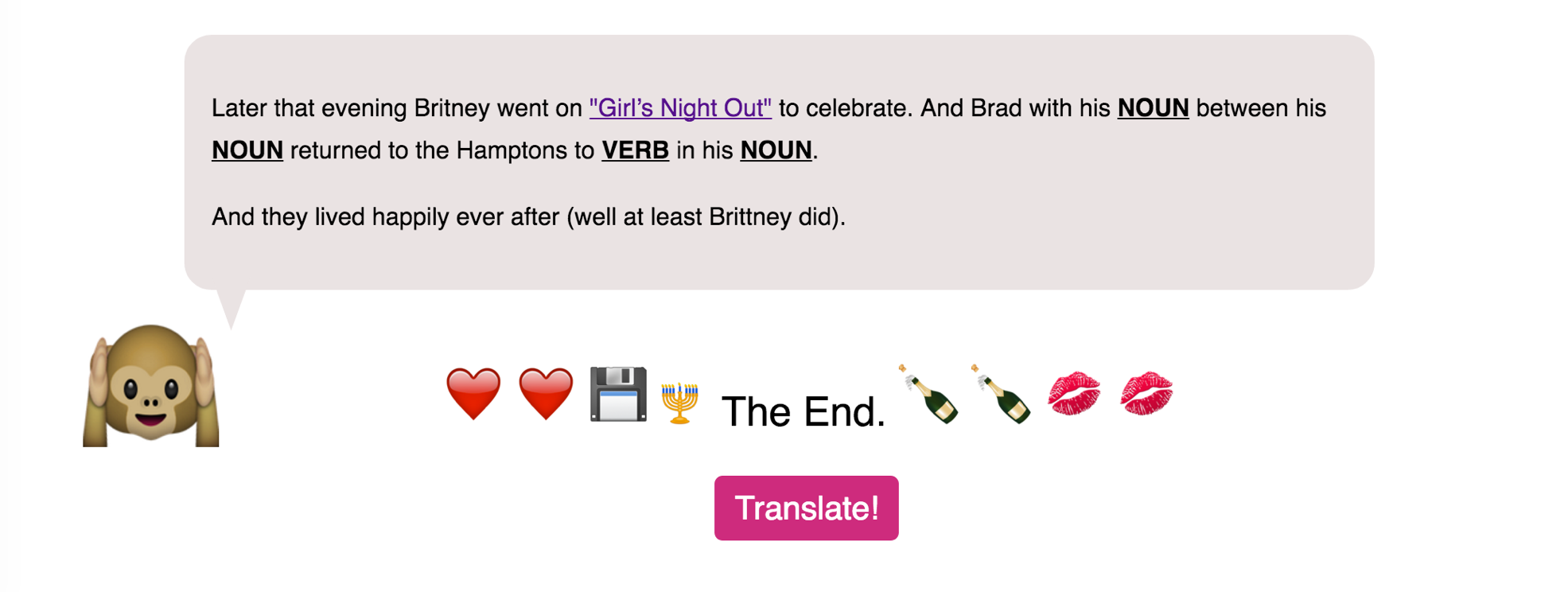
Can't wait to check it out?
My reflections, aka the Zen of Code
1
The best design comes from multi-disciplinary teams
There seem to be so many stories of developers and designers at odds with each other, but this project made it even clearer why we all need to work together - only then can we inspire each other to make the best product.
3
There is nothing like seeing your creation come to life
Even the most beautiful design is still just flat. It's not dynamic. It doesn't have any functionality. When you build a site with your own two hands and see it functioning, it's an amazing feeling. I have been a writer, artist, and filmmaker and believe that, like those mediums, code is truly an art.